1. Call Stack
The call stack is a vital part of JavaScript’s execution model. It keeps track of function calls, ensuring they are executed in the correct order. When a function is called, it’s pushed onto the stack, and when it returns, it’s popped off. This stack helps maintain the program’s execution flow.
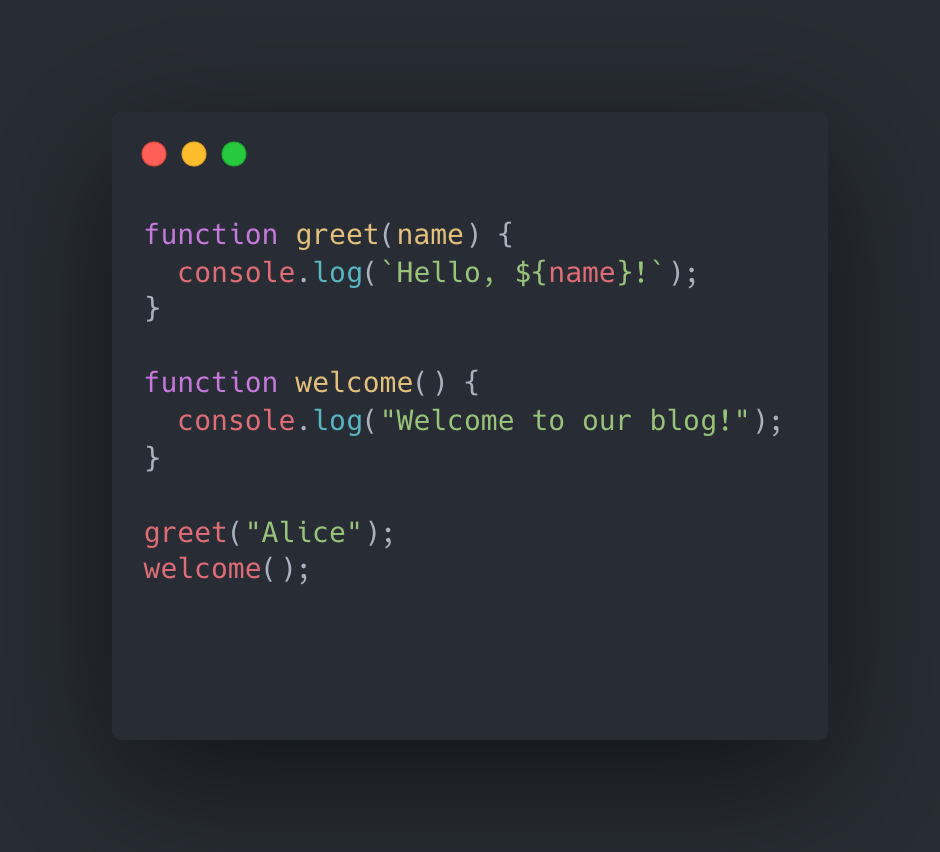
2. Primitive Types
JavaScript has six primitive data types: numbers, strings, booleans, null, undefined, and symbols. These types represent simple values and are immutable, meaning their values cannot be changed. Understanding how these types work is crucial for writing efficient and bug-free code.
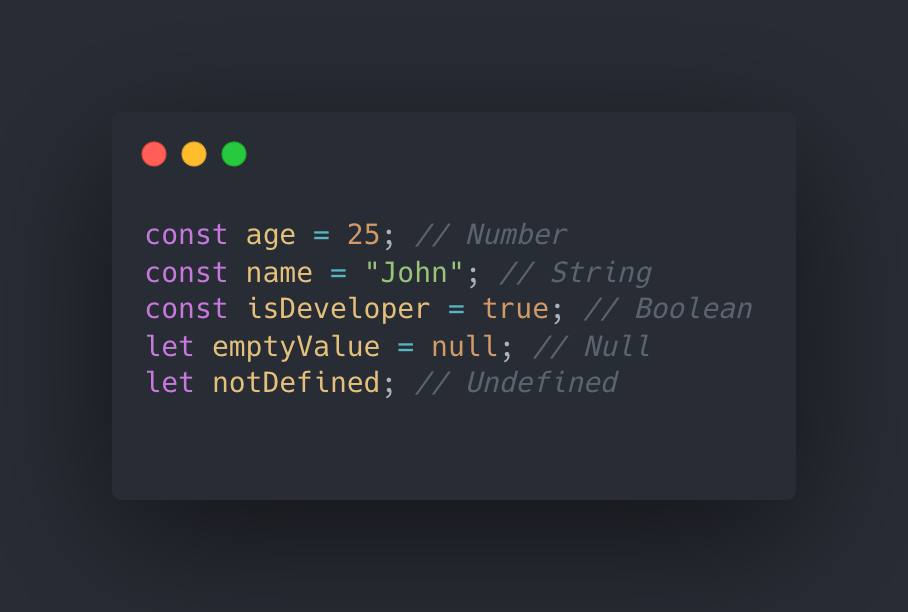
3. Value Types and Reference Types
JavaScript’s variables can hold either primitive values (value types) or references to objects (reference types). Value types, such as numbers and strings, are stored directly in memory. Reference types, on the other hand, store a reference to the actual object in memory. Understanding the difference is essential to avoid unexpected behaviors when working with objects.
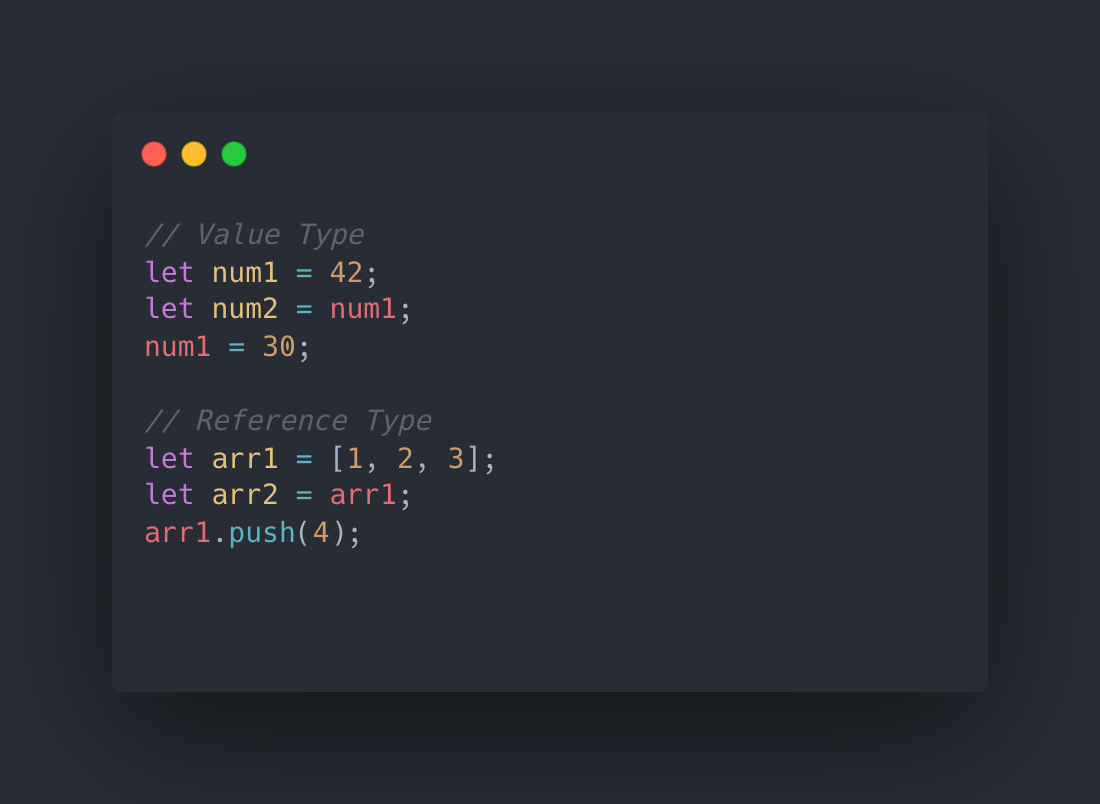
4. Type Coercion
JavaScript performs type coercion when comparing different data types. It’s crucial to understand how JavaScript coerces types in operations like comparisons and arithmetic. Implicit and explicit type coercion can lead to unexpected results, so it’s important to be aware of these behaviors.
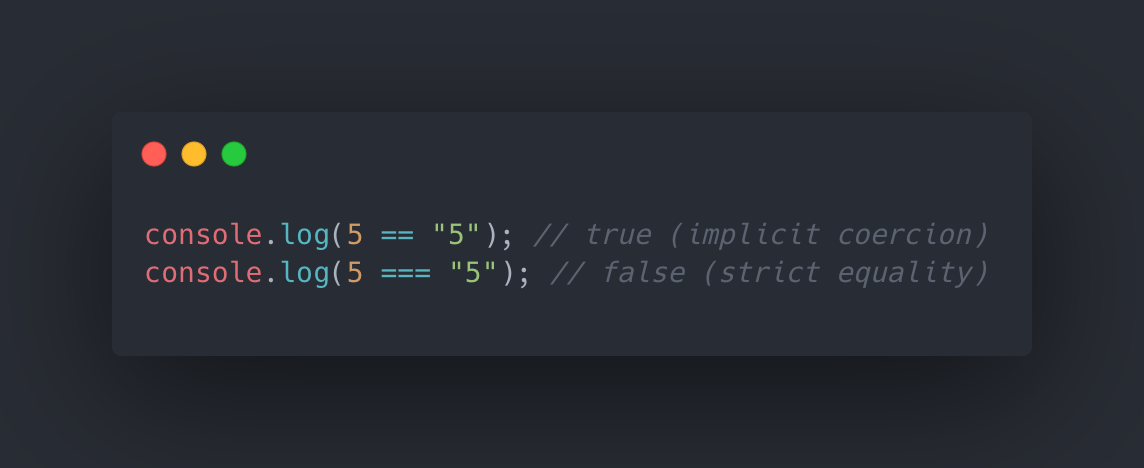
5. == vs === vs typeof
JavaScript provides two equality operators: ==
(loose equality) and ===
(strict equality). It’s essential to grasp the difference between them. Additionally, the typeof
operator helps determine the data type of a value, which is valuable when working with dynamic data.
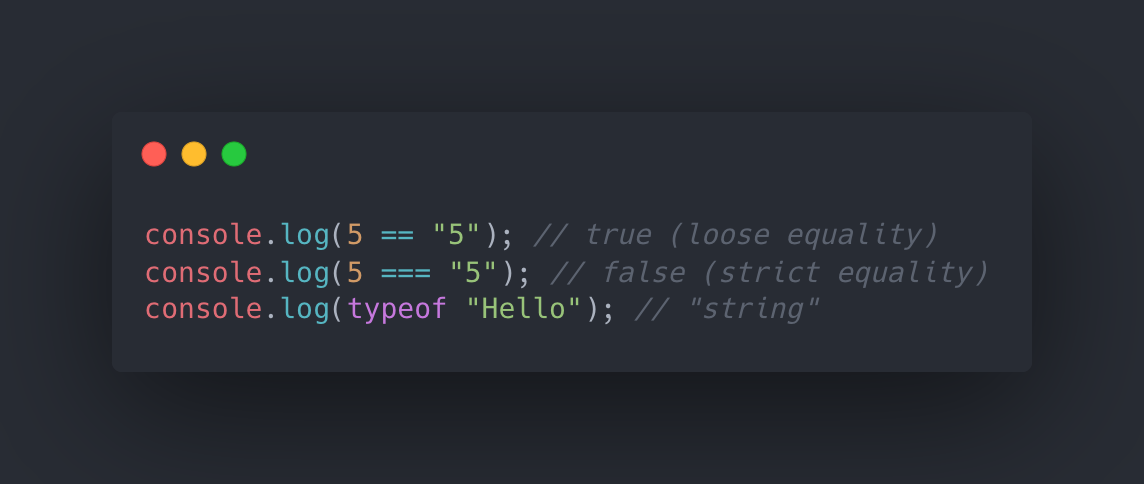
6. Scope: Function, Block, and Lexical
JavaScript features various scopes, including function scope, block scope (introduced with let
and const
), and lexical scope. Function scope means variables declared within a function are only accessible within that function. Block scope, introduced with ES6, allows variables to be scoped within blocks (e.g., if statements or loops). Lexical scope refers to how nested functions can access variables from their containing scope.
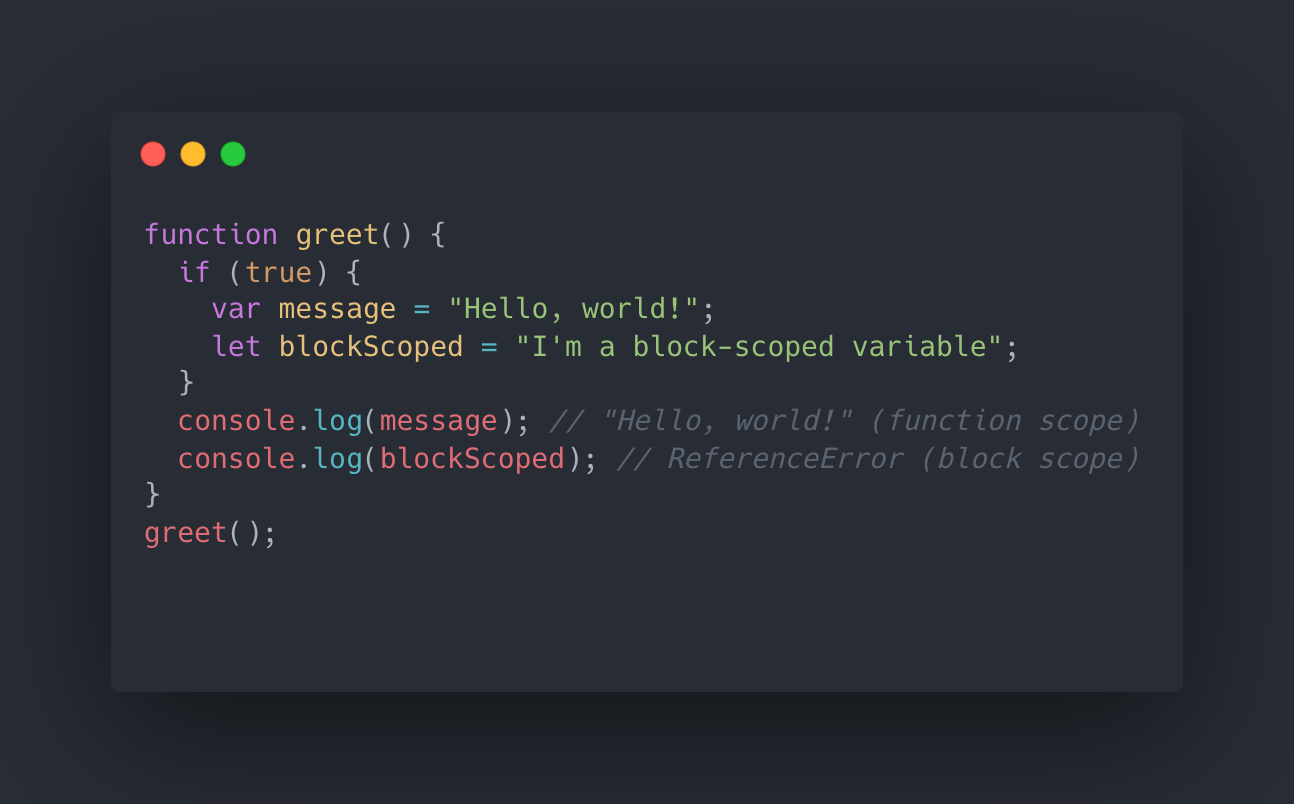
7. Expression vs Statement
In JavaScript, code can be categorized into expressions and statements. Expressions produce values, while statements perform actions. Understanding the difference between the two is essential for writing efficient and readable code.
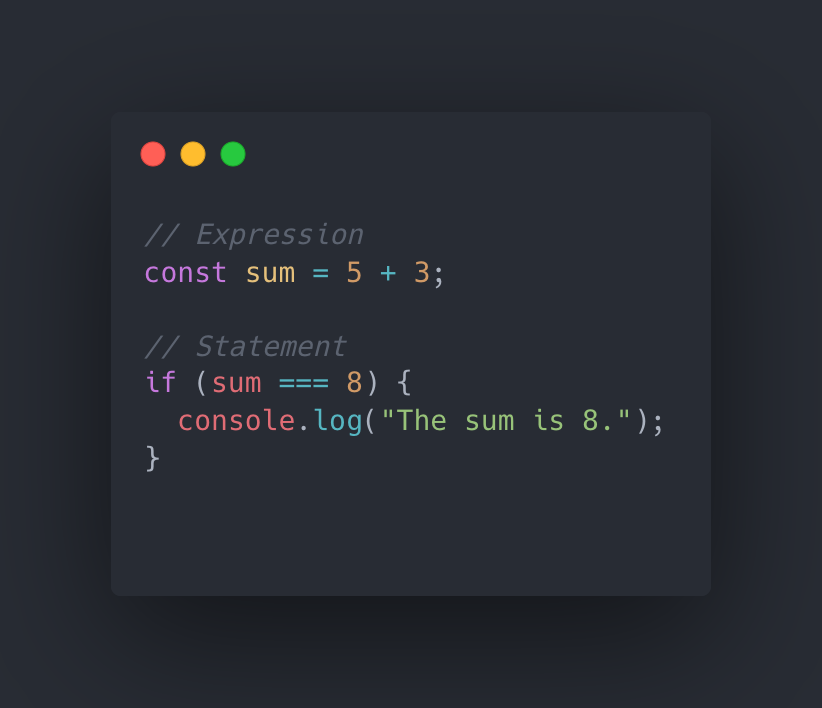
8. IIFE, Modules, and Namespaces
Immediately Invoked Function Expressions (IIFE) are self-executing functions often used to encapsulate code. ES6 introduced modules, allowing you to organize your code into reusable and maintainable pieces. Namespaces are a way to avoid naming conflicts in large codebases.
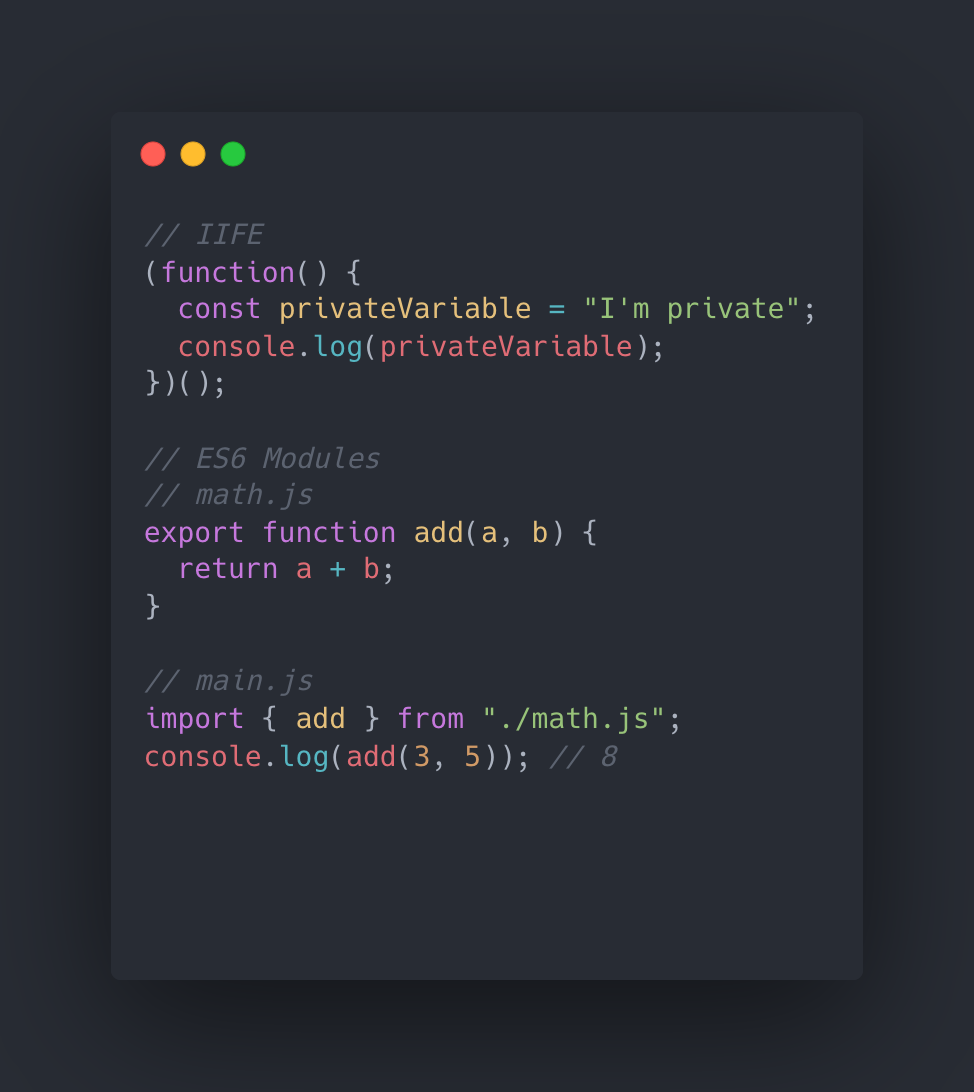
9. Message Queue and Event Loop
Understanding asynchronous JavaScript is vital. JavaScript uses a message queue and event loop to handle asynchronous operations. This concept is essential when working with timers, AJAX requests, and other asynchronous tasks.
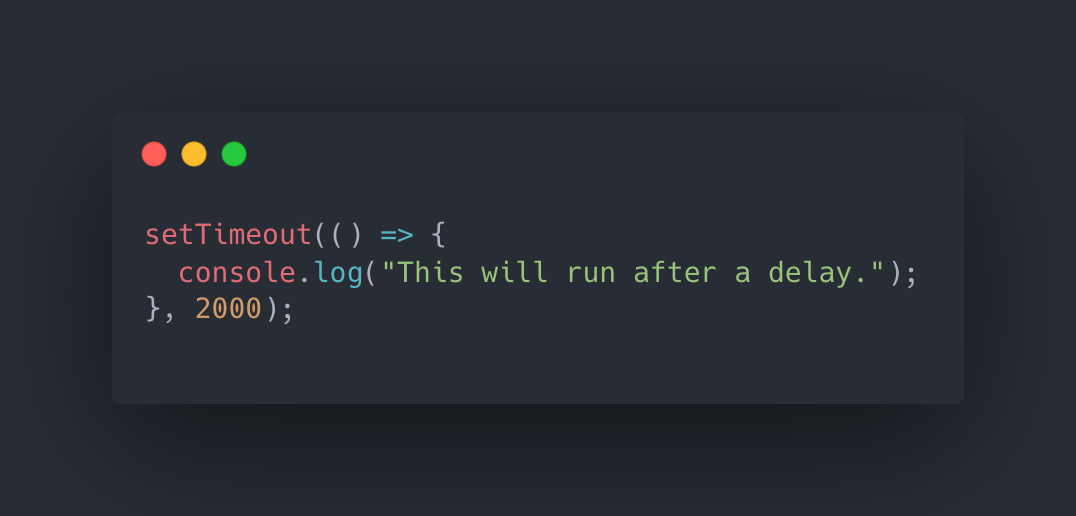
10. setTimeout, setInterval, and requestAnimationFrame
These timing functions are crucial for controlling when and how specific code executes. setTimeout
executes a function after a specified delay, setInterval
repeatedly executes a function at intervals, and requestAnimationFrame
is used for smooth animations.
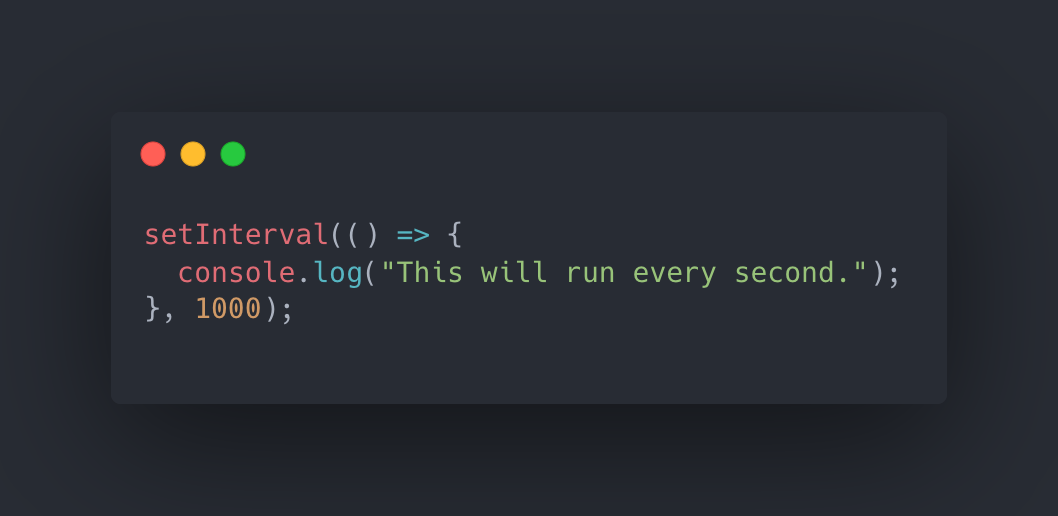